Tutorials
The following tutorials provide an in-depth introduction into the Keysight’s Quantum Control System programming framework.
Key Components
The Keysight’s Quantum Control System library consists of the following key components:
Programs: A program is represented by the
Program
class that allows users to define a set of instructions to be executed in order. The instructions can be defined at the gate, Hamiltonian, or pulse level with relative timing or the pulse/hardware level with absolute timing.Targets: Individual channels of the M5000 modules are represented by the
Channels
class, and can be mapped to the channels in the AWG and digitizer modules using theChannelMapper
class. Qudits can be defined using theQudits
class. Mapping between qudits and channels is accomplished usingBaseLinker
objects. See Introduction to linkers for more information.Operations: The fundamental instructions on AWG channels are
HardwareOperation
s, such asRFWaveform
s,DCWaveform
s andDelay
s. Quantum instructions, such asGate
s andHamiltonian
s, are specified onQudits
s.Executor: The
Executor
compiles programs and can run them on the Keysight’s Quantum Control System hardware. It is instantiated with a list ofPass
objects for the compilation. Theexecute
method iterates through those passes and applies them sequentially. Running a program on hardware is accomplished by a pass as well, namely theHclBackend
pass.Results/Analysis: Raw trace and IQ data acquired through the digitizer channels are stored in a database managed by QCS and can be accessed through the program.
The following short example demonstrates how these components work together:
import keysight.qcs as qcs
# set the following variable to True when connected to hardware
run_on_hw = False
# define abstract channels for the AWG and digitizer
awg = qcs.Channels(1, "awg")
dig = qcs.Channels(1, "dig")
# instantiate a program with a waveform and an acquisition
program = qcs.Program()
gauss = qcs.RFWaveform(50e-9, qcs.GaussianEnvelope(), 0.2, 5e9)
program.add_waveform(gauss, awg)
program.add_acquisition(500e-9, dig)
# the channel mapper maps our channels to physical channels
mapper = qcs.ChannelMapper()
awg_address = qcs.Address(chassis=1, slot=1, channel=1)
dig_address = qcs.Address(1, 2, 1)
mapper.add_channel_mapping(awg, awg_address, qcs.InstrumentEnum.M5300AWG)
mapper.add_channel_mapping(dig, dig_address, qcs.InstrumentEnum.M5200Digitizer)
# specify a local oscillator frequency for the AWG
mapper.set_lo_frequencies(awg_address, 4.9e9)
# Execute the program
if run_on_hw:
program_executed = qcs.Executor(qcs.HclBackend(mapper)).execute(program)
# optional: save program and data to a file
program_executed.to_hdf5("my_program.hdf5")
Guides
The guides below give a detailed introduction to the Keysight’s Quantum Control System
Program
structure and the associated timing model,
how to execute programs on hardware, the available
HardwareOperation
s, and how to traverse between
different layers of abstraction using
Linkers
s.
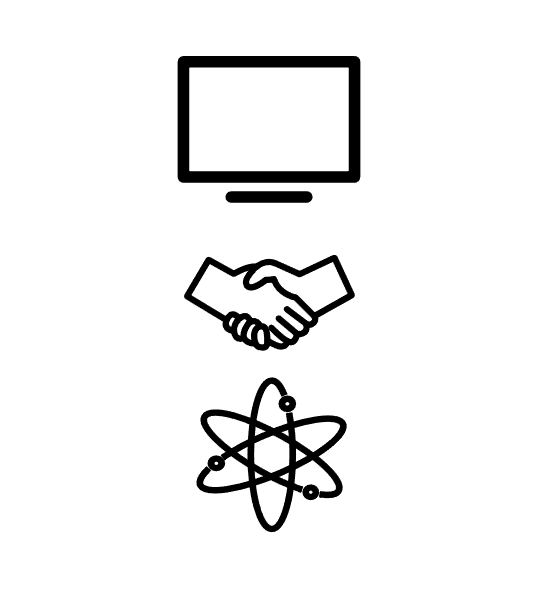
Working with QCS hardware
These tutorials provide an in-depth introduction of the typical workflow in
the Keysight’s Quantum Control System library including relevant elements such as the
ChannelMapper
and the
CalibrationSet
and how to connect to the
Keysight hardware.
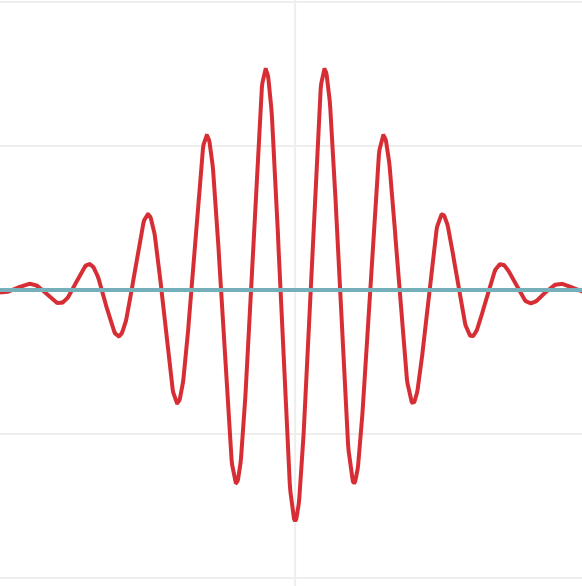
Waveform representations
These tutorials provide an overview of the built-in pulse envelopes and user-defined arbitrary envelopes and demonstrates how they can be implemented to manipulate the RF output.
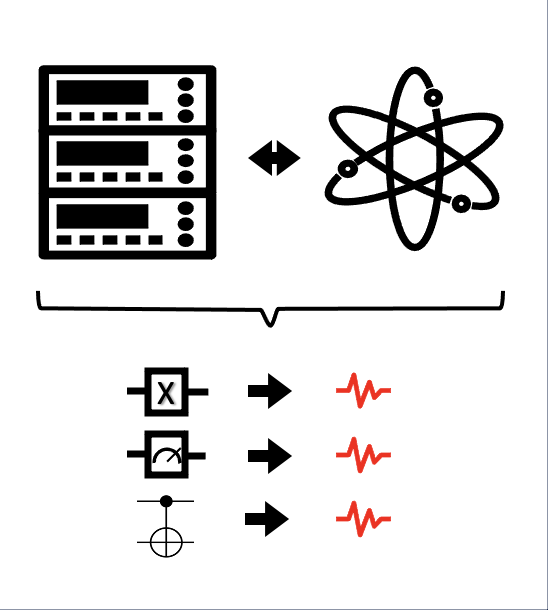
Program compilation
These tutorials outline how users can manage different abstraction layers in
their programs through Linkers
.