Welcome to Keysight’s Quantum Control System
The Keysight’s Quantum Control System (QCS) library provides an interface to M5000 Series PXI modules and enables users to easily program quantum processors at different levels of abstraction. This includes control from the level of gates or circuits, as well as direct control of the arbitrary waveform generators (AWGs), downconverters, and digitizers.
Operating a quantum processor involves many different tasks, each with their own terminology and skillset. These tasks are most naturally defined relative to one of the following abstraction levels:
Discrete operations (gates) applied to abstract qudits;
Continuous operations (parametric gates using Hamiltonians) acting on abstract qudits; or
Continuous-time voltages of physical instruments.
Keysight’s Quantum Control System enables us to work at each of these levels with a common interface to enable cutting-edge quantum processing.
Waveform programs
A simple program at the waveform level can be written in a few short lines:
import keysight.qcs as qcs
# define abstract channels for the two AWGs and two digitizers
awg = qcs.Channels(range(2), "awg")
dig = qcs.Channels(range(2), "dig")
# instantiate a program and add a waveform to play on the AWG channels
program = qcs.Program()
# create a Gaussian pulse with 50 ns duration, an amplitude of 20% of the channel's
# maximum and a frequency of 5 GHz:
gauss = qcs.RFWaveform(50e-9, qcs.GaussianEnvelope(), 0.2, 5e9)
# add this waveform to both channels
program.add_waveform(gauss, awg)
# add a wait period to the second channel followed by another pulse
program.add_waveform(qcs.Delay(200e-9), awg[1], new_layer=True)
program.add_waveform(gauss, awg[1])
# add a 500 ns acquisition on the digitizer channel after the AWG pulses
program.add_acquisition(500e-9, dig, new_layer=True)
program.draw()
Program
Program
|
||||||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Layer #0
Layer #0
|
Layer #1
Layer #1
|
Layer #2
Layer #2
|
||||||||||||||||||||||||||||
|
|
RFWaveform on ('awg', 0)
Parameters
|
||||||||||||||||||||||||||||
|
RFWaveform on ('awg', 1)
Parameters
|
Delay on ('awg', 1)
Parameters
|
RFWaveform on ('awg', 1)
Parameters
|
|||||||||||||||||||||||||||
|
|
Acquisition on ('dig', 0)
Parameters
|
||||||||||||||||||||||||||||
|
Acquisition on ('dig', 1)
Parameters
|
Quantum Circuits
Similarly, a simple quantum circuit program can be written as:
qudits1 = qcs.Qudits(range(2), "xy_qudits")
qudits2 = qcs.Qudits(2, "other")
program = qcs.Program()
program.add_gate(qcs.GATES.x, qudits1[0])
program.add_gate(qcs.GATES.cx, (qudits1[0], qudits2[0]))
program.add_gate(qcs.GATES.y, qudits2, new_layer=True)
program.add_gate(qcs.GATES.cx, (qudits1[1], qudits2[0]))
program.add_measurement(qudits1[1], new_layer=True)
program.add_measurement(qudits2, new_layer=True)
program.draw()
Program
Program
|
|||||||||||||||||||||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Layer #0
Layer #0
|
Layer #1
Layer #1
|
Layer #2
Layer #2
|
Layer #3
Layer #3
|
Layer #4
Layer #4
|
Layer #5
Layer #5
|
||||||||||||||||||||||||||||||||||||||||
|
|
X
Gate X on ('xy_qudits', 0)
Matrix:
|
CX
Gate CX on (('xy_qudits', 0), ('other', 2))
Matrix:
|
||||||||||||||||||||||||||||||||||||||||||
|
CX
Gate CX on (('xy_qudits', 1), ('other', 2))
Matrix:
|
Measure on ('xy_qudits', 1)
Parameters
|
|||||||||||||||||||||||||||||||||||||||||||
|
|
CX
Gate CX on (('other', 2), ('xy_qudits', 0))
Matrix:
|
Y
Gate Y on ('other', 2)
Matrix:
|
CX
Gate CX on (('other', 2), ('xy_qudits', 1))
Matrix:
|
Measure on ('other', 2)
Parameters
|
Guides
The following pages provide a detailed guide for how to use the Keysight’s Quantum Control System framework. For a quick start, users can jump right to our Running a program on hardware tutorial. The tutorials introduce the basic types and show how the pieces fit together, and the applications show the full workflow for concrete applications.
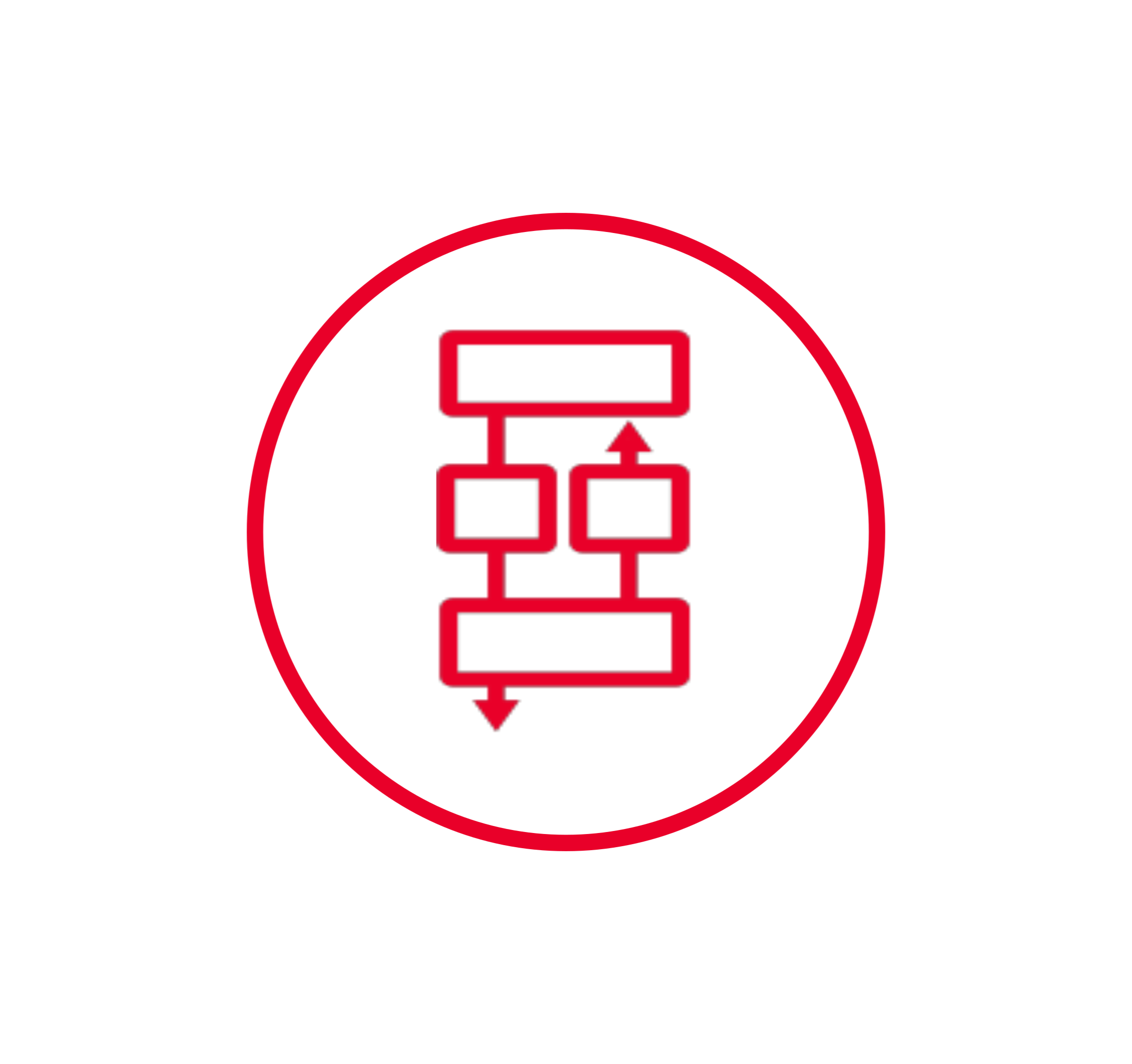
Tutorials
Check out our collection of tutorials to understand the main functionalities offered by Keysight’s Quantum Control System.
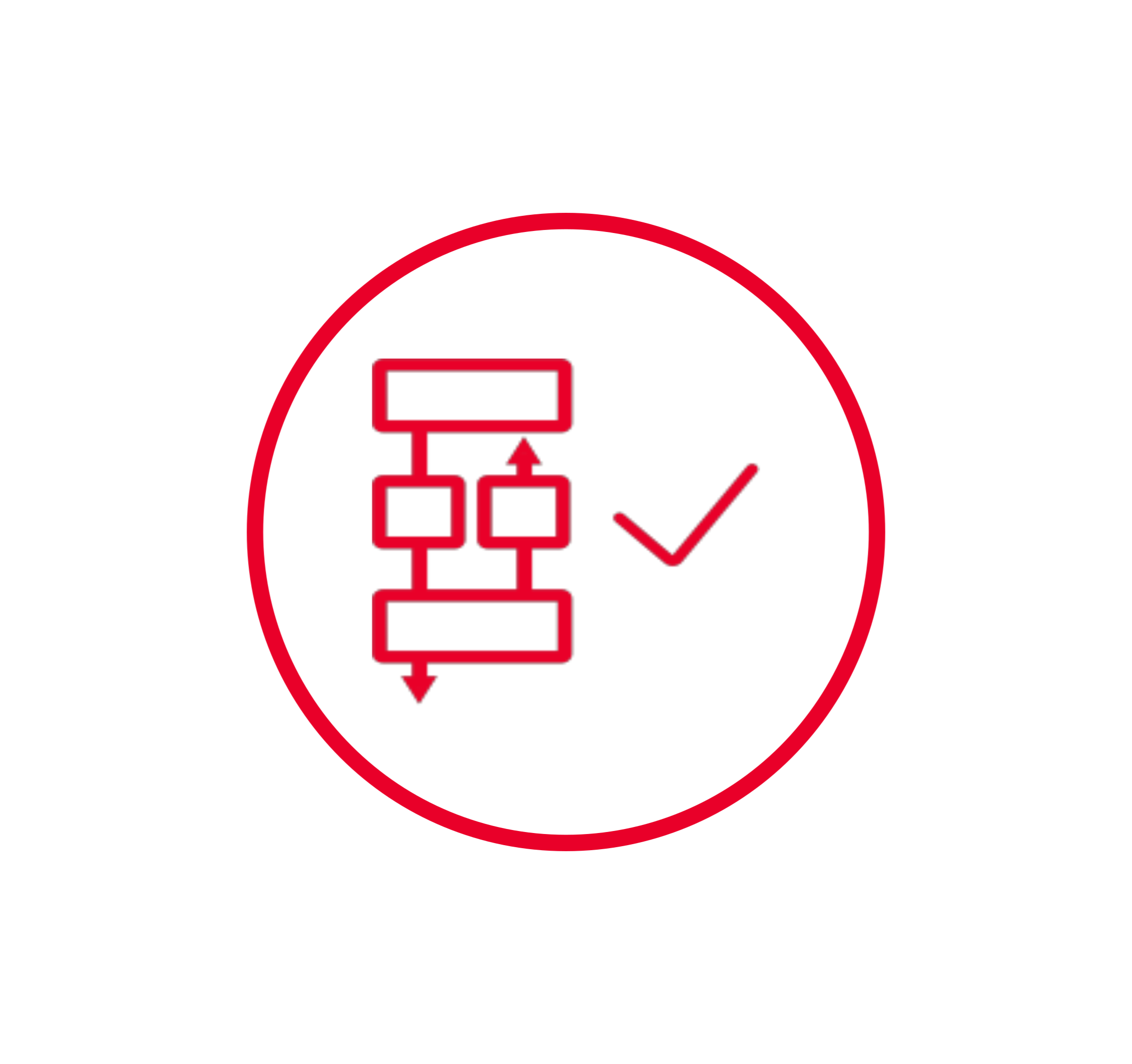
Applications
Learn how to use Keysight’s Quantum Control System’s functionalities by looking at experimentally-relevant examples.